Introduction to NestJS: A Modern Backend Framework
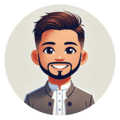
Full Stack Developer | SEO
NestJS has emerged as a go-to framework for building scalable and maintainable server-side applications. Inspired by Angular’s architecture and powered by TypeScript, it combines flexibility with structure—making it ideal for both small projects and enterprise-grade apps. In this guide, you’ll learn why NestJS is loved by developers and how it simplifies backend development.
What is NestJS?
NestJS is a progressive backend framework for Node.js that leverages TypeScript (or JavaScript) to build efficient and scalable applications. Unlike minimalist frameworks like Express.js, NestJS provides an opinionated architecture inspired by Angular, emphasizing:
Modularity: Code organized into reusable modules.
Dependency Injection: Clean, testable, and decoupled components.
Out-of-the-box Tools: Built-in support for REST/GraphQL APIs, WebSockets, and microservices.
It’s designed for developers who value structure without sacrificing flexibility.
Prerequisites for Learning NestJS
Before diving into NestJS, ensure you’re familiar with:
Node.js & npm/npx: Basics of setting up a Node.js project.
TypeScript (Optional): Recommended for better type safety.
HTTP Concepts: Understanding of REST APIs, headers, and status codes.
JavaScript OOP: Classes, decorators, and inheritance.
No prior Angular knowledge is required, but it helps!
How Does NestJS Work?
NestJS follows a modular architecture where every component has a specific role:
Modules: Building blocks that group related features (e.g.,
UserModule
,AuthModule
).Controllers: Handle incoming HTTP requests and route them to services.
Providers: Reusable services (e.g., database logic, utilities) injected into controllers.
Decorators: Simplify code with annotations like
@Controller()
or@Get()
.
Under the hood, NestJS uses Express.js or Fastify to handle HTTP requests, giving you the best of both worlds: structure and performance.
Key Features of NestJS
Modular Architecture: Organize code into independent, reusable modules.
TypeScript-First: Built-in type safety for fewer runtime errors.
CLI Tool: Generate modules, controllers, and services with one command.
Extensible: Integrate databases (TypeORM, Prisma), authentication (Passport.js), and more.
Enterprise-Ready: Supports microservices, WebSockets, and GraphQL out of the box.
Advantages of NestJS
✅ Scalability: Perfect for large teams and complex projects.
✅ Maintainable Code: Clear structure reduces spaghetti code.
✅ TypeScript Benefits: Autocompletion, static typing, and better documentation.
✅ Rich Ecosystem: Plugins for Swagger, testing (Jest), and deployment (Docker).
✅ Active Community: Strong support from enterprises and open-source contributors.
Disadvantages of NestJS
❌ Learning Curve: Requires understanding of Angular-like concepts (modules, DI).
❌ Overkill for Small Projects: Better suited for medium-to-large apps.
❌ Boilerplate: More setup compared to Express.js.
When Should You Use NestJS?
Building REST/GraphQL APIs for startups or enterprises.
Working on microservices or real-time apps (e.g., chat apps).
Needing a structured framework for team collaboration.
What’s Next?
Now that you understand NestJS’s core concepts, the next step is to set up your first project. In upcoming articles, we’ll explore Modules, Controllers, and Services in detail—stay tuned!